- Markov
- 文章數 : 2
積分 : 6
注冊日期 : 2020-05-21
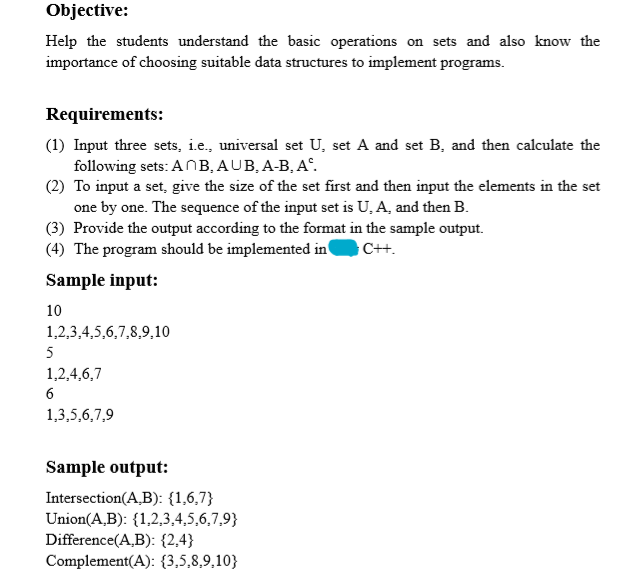
Objective: Help the students understand the basic operations on sets and also know the importance of choosing suitable data structures to implement programs. Requirements: (1) Input three sets, i.e., universal set U, set A and set B, and then calculate the following sets: ANB,AUB.A-B, A. (2) To input a set, give the size of the set first and then input the elements in the set one by one. The sequence of the input set is U. A, and then B. (3) Provide the output according to the format in the sample output. (4) The program should be implemented in C++. Sample input: 10 1,2,3,4,5,6,7,8,9,10 5 1,2,4,6,7 6 1,3,5,6,7,9 Sample output: Intersection(A,B): {1,6,7} Union(A,B): {1,2,3,4,5,6,7,9} Difference(A,B): 2,4} Complement(A): {3,5,8,9,10}
- Christopher N.Contributor
- 文章數 : 16
積分 : 18
注冊日期 : 2020-05-01
#include
#include
#include
#include
#include
#include
using namespace std;
// utility function which takes a string as input
// and returns a vector containing the numbers
vector < int > stringToVec(string str) {
vector < int > result;
stringstream ss(str);
for (int i; ss >> i;) {
result.push_back(i);
if (ss.peek() == ',')
ss.ignore();
}
sort(result.begin(), result.end());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// intersection of two vectors
vector < int > Intersection(vector < int > A, vector < int > B) {
// initialising result vector which will store
// intersection of the two vectors
vector < int > result(A.size() + B.size());
// performing set intersection
auto intersect = set_intersection(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(intersect - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// union of two vectors
vector < int > Union(vector < int > A, vector < int > B) {
// initialising result vector which will store
// union of the two vectors
vector < int > result(A.size() + B.size());
// performing set union
auto union_ = set_union(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(union_ - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// difference of first vector with second vector
vector < int > Difference(vector < int > A, vector < int > B) {
// initialising result vector which will store
// difference of the two vectors
vector < int > result(A.size() + B.size());
// performing set difference
auto difference = set_difference(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(difference - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// complement of second vector w.r.t. first vector
vector < int > Complement(vector < int > U, vector < int > A) {
// initialising result vector which will store
// complement of the two vectors
vector < int > result(U.size() + A.size());
result = Difference(U, A);
// returning the result
return result;
}
int main() {
// declaring variable to store the sets
int U_size, A_size, B_size;
string U_, A_, B_;
// taking user input
cin >> U_size;
cin >> U_;
cin >> A_size;
cin >> A_;
cin >> B_size;
cin >> B_;
vector < int > U(U_size), A(A_size), B(B_size);
U = stringToVec(U_);
A = stringToVec(A_);
B = stringToVec(B_);
cout << endl;
cout << "Intersection(A, B): {";
vector < int > g1 = Intersection(A, B);
for (auto i = g1.begin(); i != g1.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Union(A, B): {";
vector < int > g2 = Union(A, B);
for (auto i = g2.begin(); i != g2.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Difference(A, B): {";
vector < int > g3 = Difference(A, B);
for (auto i = g3.begin(); i != g3.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Complement(A): {";
vector < int > g4 = Complement(U, A);
for (auto i = g4.begin(); i != g4.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
return 0;
}
#include
#include
#include
#include
#include
using namespace std;
// utility function which takes a string as input
// and returns a vector containing the numbers
vector < int > stringToVec(string str) {
vector < int > result;
stringstream ss(str);
for (int i; ss >> i;) {
result.push_back(i);
if (ss.peek() == ',')
ss.ignore();
}
sort(result.begin(), result.end());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// intersection of two vectors
vector < int > Intersection(vector < int > A, vector < int > B) {
// initialising result vector which will store
// intersection of the two vectors
vector < int > result(A.size() + B.size());
// performing set intersection
auto intersect = set_intersection(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(intersect - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// union of two vectors
vector < int > Union(vector < int > A, vector < int > B) {
// initialising result vector which will store
// union of the two vectors
vector < int > result(A.size() + B.size());
// performing set union
auto union_ = set_union(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(union_ - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// difference of first vector with second vector
vector < int > Difference(vector < int > A, vector < int > B) {
// initialising result vector which will store
// difference of the two vectors
vector < int > result(A.size() + B.size());
// performing set difference
auto difference = set_difference(A.begin(), A.end(), B.begin(), B.end(), result.begin());
result.resize(difference - result.begin());
// returning the result
return result;
}
// function which takes two vectors and return a vector containing
// complement of second vector w.r.t. first vector
vector < int > Complement(vector < int > U, vector < int > A) {
// initialising result vector which will store
// complement of the two vectors
vector < int > result(U.size() + A.size());
result = Difference(U, A);
// returning the result
return result;
}
int main() {
// declaring variable to store the sets
int U_size, A_size, B_size;
string U_, A_, B_;
// taking user input
cin >> U_size;
cin >> U_;
cin >> A_size;
cin >> A_;
cin >> B_size;
cin >> B_;
vector < int > U(U_size), A(A_size), B(B_size);
U = stringToVec(U_);
A = stringToVec(A_);
B = stringToVec(B_);
cout << endl;
cout << "Intersection(A, B): {";
vector < int > g1 = Intersection(A, B);
for (auto i = g1.begin(); i != g1.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Union(A, B): {";
vector < int > g2 = Union(A, B);
for (auto i = g2.begin(); i != g2.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Difference(A, B): {";
vector < int > g3 = Difference(A, B);
for (auto i = g3.begin(); i != g3.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
cout << "Complement(A): {";
vector < int > g4 = Complement(U, A);
for (auto i = g4.begin(); i != g4.end(); ++i)
cout << * i << ",";
cout << "\b";
cout << "}\n";
return 0;
}
這個論壇的權限:
您 無法 在這個版面回復文章